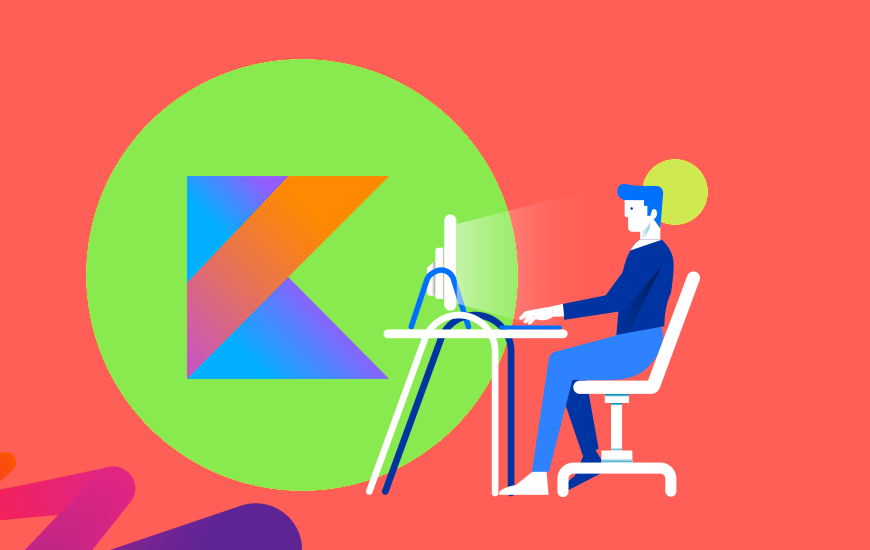
The Android programming language, Kotlin has gained a ton of popularity over a short period of time. Today, Kotlin is being used in a majority of sectors which also includes its application in mobile app development and server-side systems. Kotlin is basically a statically typed JVM language that was developed by JetBrains i.e. makers of IntelliJ IDEA.
Nowadays the link between a Java developer and Kotlin is crucial in order to deliver digital products that are efficient and innovative. This programming language is built upon another language, Java that further provides it with many features like:
- Data classes
- Null safety
- Functional Concepts
- Operator overloading
Apart from the above-mentioned features, there are more features like extensions, smart casts and many more features that can lead to a better Android app development process.
So in this article, we will be stating the things about Kotlin that every Java developer should know of. Since its inception, Kotlin has been highly compared to Java and there are various useful features of Kotlin language that are currently not available in Java. Here, we will be discussing the list of those Kotlin features that the Java developers should be aware of.
Kotlin Facts All Java Developers Should Know
Below is a list of some of the top things that every Java developer should know about Kotlin:
1. Null Safety
Every now and then, there is something called NPE that every Java developer has to face at some point while working on the Java programming language. The NullPointerException i.e. NPE can also be referred to as an element used to evaluate software quality. In JVM, this exception is used to avoid the abortion of the program by the operating system itself.
Also, this prevention is limited to the run-time of the software but on the other hand, the app developers would prefer to avoid such situations during the compilation time. This situation in Java is somewhat unnecessary as it doesn’t provide any information about null safe.
On the other hand, Kotlin offers the feature of null safety for the Java developers at the initiation stage. This is done by mentioning whether the values will be assigned to the null factor or not. Here, null safety is provided by the compiler, which also reduces the risk of encountering run-time Null Point Exceptions.
For example:
data class User(val name: String,
val surname: String,
val age: Int?,
val lastSeen: LocalDate? = null)
fun main(args: Array) {
User(
name =”John”,
surname = “Smith”,
age = null // assignment required, but ‘null’ allowed
// ‘lastSeen’ can be ommitted, defaults to ‘null’
)
}
view raw
2. Extension Functions
The Extension Functions can also be referred to as the useful Standard Library Functions that are offered by Kotlin programming language. When it comes to functional programming the functions are the basics of the object-oriented languages that require classes as well as objects for their operation.
In Java, developers can only introduce a new functionality into an existing class when that particular class is expanding and that might be the case always. But Kotlin offers the facility for extension functions to the Java developers so that new functionalities can be smoothly added into existing classes.
Apart from this, the standard library of Kotlin also provides an entire set of powerful standard functions which can be further added to any class by default.
For example, the ‘apply’ function
The ‘apply’ function can be stated as a function that is similar to ‘also’ function as it provides the object’s original reference. To get over the issue of scoped ‘this’ the Java developers can proceed with AssertJ’s soft assertions as shown in the code below.
@Test
fun `test using soft assertions`() {
val subject = User(age = 18, name = “John”)
SoftAssertions().apply {
assertThat(subject.age).isPositive()
assertThat(subject.name).isNullOrEmpty()
}.assertAll()
}
The function ‘apply’ can come in quite handy when a large number of function calls are needed to be applied to a particular object.
3. Collections and Streams
In Java programming language, processing object collections have always been an essential part of the process of app development. Mainly, the translation of objects with different representations takes place here. Although Java 8 introduced streams to eliminate the issues regarding functional programming.
- first(), last() – Extracts the first or last element matching the given predicate function.
- single() – Extracts the only element matching the given predicate, failing if more elements match.
- associate(), associateBy() – Transforms from a list to map entry by associating keys with values.
The above-mentioned Kotlin functions tend to return the immutable collections by default. This further ensures that the side-effects faced in Java are applicable in this programming language.
4. Named Parameters
The named parameters are one of the most common differences that app developers can find between both of the programming languages. In the case of Java, users are not allowed to explicitly name the parameters in their function calls, making them completely rely on positional parameters.
Whereas, in the case of Kotlin, developers have two options to pass parameters that are either by option or by name. But both of these methods can not be applied at the same time. In addition to this, the trending programming language also offers a default value declaration to enable callers to omit the parameters.
For example:
fun mixedRequiredAndOptional(x: Int, str: String = “Hello”, y: Int) {
…
}
fun main(args: Array) {
mixedRequiredAndOptional(1, 2) // won’t compile, ‘str’ missing
mixedRequiredAndOptional(x = 1, y =2) // will compile, ‘str’ is optional
}
view raw
When compared to other programming languages, kotlin provides no limitation upon the use of mixed style parameters when it comes to a function declaration.
5. Immutability
In the functional programming languages, the immutability is a feature that is required to avoid some of the side-effects caused by the functions. Here, Java can only offer a limited amount of support to ensure that the software is immutable during the time of compilation.
Here the code is basically not allowed to alter the state outside its scope, this leads to being free of the side-effects. To overcome this issue, kotlin provides the high-end compiler support that can easily distinguish between the properties and value that are mutable or immutable in nature.
For example:
val user = User(“John”)
user = User(“Another”) // won’t compile, val is immutable
user.name = “Another” // won’t compile, if ‘name’ is val
user.lastSeen = LocalDate.now() // will compile, if ‘lastSeen’ is var
It is important to deal with this situation because multiple equally typed properties of an object will contribute to an error-prone software with object construction or duplication.
6. String Interpolation
Strings in Java can sometimes be hard to deal with and this includes the use of plus operators, StringBuffer and String.format(). Let’s take the example of, using the plus operator and StringUtil(s) classes for reference. Kotlin offers the solution to this issue by simplifying the process of string handling with string interpolation.
With string interpolation, the expressions in the code can directly be embedded into strings by using the expression ${expr} for the variables that are simple. In the case of referring to the on-string objects, the toString() is automatically called and that also helps in minimizing the boiler-plating occurrence in the code.
For example:
data class Coordinate(val lat: Double,
val lng: Double)
fun main(args: Array) {
val coordinates = listOf(
Coordinate(42.3, 12.3),
Coordinate(52.1, 9.8)
)
val json = “””
{
“id”: “${UUID.randomUUID()}”,
“coordinates”: [
${coordinates.joinToString {
“””
[
“lat”: ${it.lat},
“lng”: ${it.lng}
]
“””
}}
]
}
“””
println(json)
}
When dealing with iterations, the string interpolation feature in Kotlin can be very helpful as it can be iterated over collections. The Java developers can even produce some partial strings as per the elements that can be further joined to form an entire string using the StringBuffer as shown in the above code.
7. Singletons
As we already know that programming language, Kotlin has a keyword known as the object whose job is to enable an object to be defined similarly to the class. According to that, the object only exists as a single instance which can be further used to create singletons as the feature is not limited.
This whole concept can be said as a strong extension to the enums, interfaces and even classes that are available in the Java language. This is because the elements in this domain model are also inherently objects that may exist only once during runtime.
8. Sealed Classes & Enumerations
Another thing about Kotlin that the Java developers should know about are sealed classes and enumerations. This programming language also offers a high-level approach to regular enumerations. But on the other hand, no such support is provided by Java except for allowing enums to define properties and attributes for different instances.
For example:
sealed class Operation {
object Show: Operation()
object Hide: Operation()
data class Log(val message: String): Operation()
data class Exit(val rc: Int): Operation()
}
fun handleOp(op: Operation) {
when(op) {
Operation.Show -> println(“show”)
Operation.Hide -> println(“hide”)
is Operation.Log -> println(“log: ${op.message}”)
is Operation.Exit -> System.exit(op.rc)
}
}
It can also be said that the abstract data types are well defined in Kotlin using sealed classes. These sealed classes can also be further used in creating enumerations along with the flexible sub-classes. Here, the compiler ensures that only those classes can be directly extended (or nested) in a sealed class, can be named as a valid example of enumeration.
Final Thoughts
One of the main reasons why Kotlin is slowly gaining a lead over Java is its simplicity as a programming language. Basically, Kotlin cleans up the process by eliminating the code bloating that occurs in Java. Also, the Java developerswon’t find it difficult to switch to Kotlin as both languages are quite similar in their concepts.
And in order to make our points more clear and effective for your understanding, we have listed below some of the main advantages as well as disadvantages of both programming languages i.e. Kotlin and Java.
We hope with the help of this article, you were able to gain some valuable insights about Java developers and Kotlin in the upcoming years. Also, we would love to know your thoughts regarding this statically typed language that runs on the Java Virtual Machine.
And in case, you are interested in reading more such interesting and informative articles then make sure you click on that ‘Subscribe’ button to stay notified of all updated regarding the mobile app industry.
This article is shared by www.fortunescripts.com | A leading resource of inspired clone scripts. It offers hundreds of popular scripts that are used by thousands of small and medium enterprises.